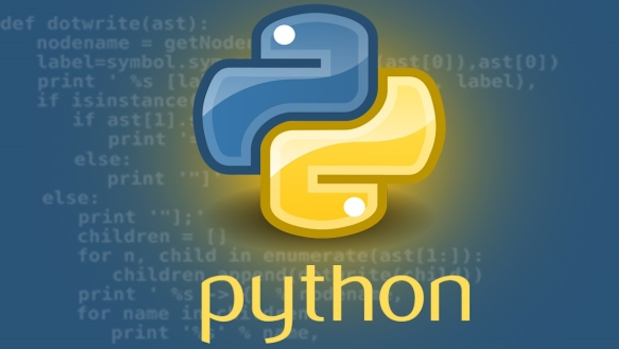
Cython: Python at the speed of C
Python has a reputation for being one of the most convenient, richly outfitted, and downright useful programming languages. Execution speed? Not so much.
Enter Cython. The Cython language is a superset of Python that compiles to C, yielding performance boosts that can range from a few percent to several orders of magnitude, depending on the task at hand. For work that is bound by Python’s native object types, the speedups will not be large. But for numerical operations, or any operations not involving Python’s own internals, the gains can be massive.
With Cython, you can skirt many of Python’s native limitations or transcend them entirely—without having to give up Python’s ease and convenience.
Compile Python to C
Python code can make calls directly into C modules. Those C modules can be either generic C libraries or libraries built specifically to work with Python. Cython generates the second kind of module: C libraries that talk to Python’s internals, and that can be bundled with existing Python code.
Cython code looks a lot like Python code, by design. If you feed the Cython compiler a Python program (Python 2.x and Python 3.x are both supported), Cython will accept it as-is, but none of Cython’s native accelerations will come into play. But if you decorate the Python code with type annotations in Cython’s special syntax, Cython will be able to substitute fast C equivalents for slow Python objects.
Note that Cython’s approach is incremental. That means a developer can begin with an existing Python application, and speed it up by making spot changes to the code, rather than rewriting the whole application from the ground up.
This approach dovetails with the nature of software performance issues generally. In most programs, the vast majority of CPU-intensive code is concentrated in a few hot spots—a version of the Pareto principle, also known as the “80/20” rule. Thus most of the code in a Python application does not need to be performance-optimised, just a few critical pieces. You can incrementally translate those hot spots into Cython, and so get the performance gains you need where it matters most. The rest of the program can remain in Python for the convenience of the developers.
How to use Cython
Consider the following code, taken from Cython’s documentation:
def f(x):
return x**2-x
def integrate_f(a, b, N):
s = 0
dx = (b-a)/N
for i in range(N):
s += f(a+i*dx)
return s * dx
This is a toy example, a not-very-efficient implementation of an integral function. As pure Python code, it’s slow, because Python must convert back and forth between machine-native numerical types and its own internal object types.
Now consider the Cython version of the same code, with Cython’s additions underscored:
cdef double f(double x):
return x**2-x
def integrate_f(double a, double b, int N):
cdef int i
cdef double s, x, dx
s = 0
dx = (b-a)/N
for i in range(N):
s += f(a+i*dx)
return s * dx
If we explicitly declare the variable types, both for the function parameters and the variables used in the body of the function (double, int
, etc.), Cython will translate all of this into C. We can also use the cdef keyword to define functions that are implemented primarily in C for additional speed, although those functions can only be called by other Cython functions and not by Python scripts. (In the above example, only integrate_f
can be called by another Python script.)
Note how little our actual code has changed. All we have done is add type declarations to existing code to get a significant performance boost.
Cython advantages
Aside from being able to speed up the code you have already written, Cython grants several other advantages:
Working with external C libraries can be faster
Python packages like NumPy wrap C libraries in Python interfaces to make them easy to work with. However, going back and forth between Python and C through those wrappers can slow things down. Cython lets you talk to the underlying libraries directly, without Python in the way. (C++ libraries are also supported.)
You can use both C and Python memory management
If you use Python objects, they are memory-managed and garbage-collected the same as in regular Python. But if you want to create and manage your own C-level structures, and use malloc/free
to work with them, you can do so. Just remember to clean up after yourself.
You can opt for safety or speed as needed
Cython automatically performs runtime checks for common problems that pop up in C, such as out-of-bounds access on an array, by way of decorators and compiler directives (e.g. @boundscheck(False)
). Consequently, C code generated by Cython is much safer by default than hand-rolled C code, though potentially at the cost of raw performance.
If you are confident you will not need those checks at runtime, you can disable them for additional speed gains, either across an entire module or only on select functions.
Cython also allows you to natively access Python structures that use the buffer protocol for direct access to data stored in memory (without intermediate copying). Cython’s memoryviews let you work with those structures at high speed, and with the level of safety appropriate to the task. For instance, the raw data underlying a Python string can be read in this fashion (fast) without having to go through the Python runtime (slow).
Cython C code can benefit from releasing the GIL
Python’s Global Interpreter Lock, or GIL, synchronises threads within the interpreter, protecting access to Python objects and managing contention for resources. But the GIL has been widely criticised as a stumbling block to a better-performing Python, especially on multicore systems.
If you have a section of code that makes no references to Python objects and performs a long-running operation, you can mark it with the with nogil
: directive to allow it to run without the GIL. This frees up the Python interpreter to do other things, and allows Cython code to make use of multiple cores (with additional work).
Cython can use Python type hinting syntax
Python has a type-hinting syntax that is used mainly by linters and code checkers, rather than the CPython interpreter. Cython has its own custom syntax for code decorations, but with recent revisions of Cython you can use Python type-hinting syntax to provide basic type hints to Cython as well.
Cython can be used to obscure sensitive Python code
Python modules are trivially easy to decompile and inspect, but compiled binaries are not. When distributing a Python application to end users, if you want to protect some of its modules from casual snooping, you can do so by compiling them with Cython. Note, though, this is a side effect of Cython’s capabilities, not one of its intended functions.
Cython limitations
Keep in mind that Cython is not a magic wand. It does not automatically turn every instance of poky Python code into sizzling-fast C code. To make the most of Cython, you must use it wisely—and understand its limitations:
Little speedup for conventional Python code
When Cython encounteres Python code it cannot translate completely into C, it transforms that code into a series of C calls to Python’s internals. This amounts to taking Python’s interpreter out of the execution loop, which gives code a modest 15% to 20% speedup by default. Note that this is a best-case scenario; in some situations, you might see no performance improvement, or even a performance degradation.
Little speedup for native Python data structures
Python provides a slew of data structures—strings, lists, tuples, dictionaries, and so on. They are hugely convenient for developers, and they come with their own automatic memory management. But they’re slower than pure C.
Cython lets you continue to use all of the Python data structures, although without much speedup. This is, again, because Cython simply calls the C APIs in the Python runtime that create and manipulate those objects. Thus Python data structures behave much like Cython-optimised Python code generally: You sometimes get a boost, but only a little. For best results, use C variables and structures. The good news is Cython makes it easy to work with them.
Cython code runs fastest when “pure C”
If you have a function in C labelled with the cdef
keyword, with all its variables and inline function calls to other things that are pure C, it will run as fast as C can go. But if that function references any Python-native code, like a Python data structure or a call to an internal Python API, that call will be a performance bottleneck.
Fortunately, Cython provides a way to spot these bottlenecks: a source code report that shows at a glance which parts of your Cython app are pure C and which parts interact with Python. The better optimised the app, the less interaction there will be with Python.
A source code report generated for a Cython application. Areas in white are pure C; areas in yellow show interaction with Python’s internals. A well-optimised Cython program will have as little yellow as possible. The expanded last line shows the C code underlying its corresponding Cython code.
Cython NumPy
Cython improves the use of C-based third-party number-crunching libraries like NumPy. Because Cython code compiles to C, it can interact with those libraries directly, and take Python’s bottlenecks out of the loop.
But NumPy, in particular, works well with Cython. Cython has native support for specific constructions in NumPy and provides fast access to NumPy arrays. And the same familiar NumPy syntax used in a conventional Python script can be used in Cython as-is.
However, if you want to create the closest possible bindings between Cython and NumPy, you need to further decorate the code with Cython’s custom syntax. The cimport
statement, for instance, allows Cython code to see C-level constructs in libraries at compile time for the fastest possible bindings.
Since NumPy is so widely used, Cython supports NumPy “out of the box.” If you have NumPy installed, you can just state cimport numpy
in your code, then add further decoration to use the exposed functions.
Cython profiling and performance
You get the best performance from any piece of code by profiling it and seeing firsthand where the bottlenecks are. Cython provides hooks for Python’s cProfile module, so you can use Python’s own profiling tools, like cProfile, to see how your Cython code performs.
It helps to remember in all cases that Cython is not magic—that sensible real-world performance practices still apply. The less you shuttle back and forth between Python and Cython, the faster your app will run.
For instance, if you have a collection of objects you want to process in Cython, do not iterate over it in Python and invoke a Cython function at each step. Pass the entire collection to your Cython module and iterate there. This technique is used often in libraries that manage data, so it’s a good model to emulate in your own code.
We use Python because it provides programmer convenience and enables fast development. Sometimes that programmer productivity comes at the cost of performance. With Cython, just a little extra effort can give you the best of both worlds.
IDG News Service
Subscribers 0
Fans 0
Followers 0
Followers